So far we have managed to create small pieces of code without much interest because very unambitious. The problem with the interpreter is that once it is closed your work is lost.
The idea of a program is to save your work to a file and then run it. This increases your productivity but has many advantages such as massive copy and paste or just collaborative work. When code is saved in an executable file, we talk about script .
Create your first python script
First of all you must create a file with the extension .py - in our example it will be named fiche.py - in the folder you want (the location does not matter).
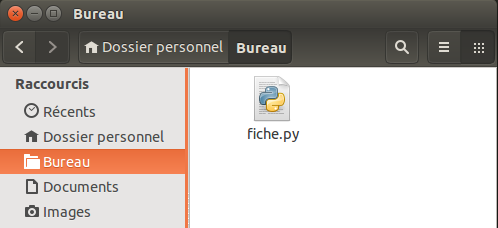
Then open the file.
Bonjour Monde
The traditional hello world is done as follows:
# coding: utf-8
print ( "Hello world" )
The first line indicates that this is python code.
The second line indicates the type of encoding used. I always advise you whatever your project and your programming language to go through UTF-8 and the third line you already know.
Run a python script
To run a python script on ubuntu you just need to run the following command:
python / path_to_your_script / form . py
Make the user interact
A program is not very interesting if the user cannot interact with it.
We will create a small script that asks the age of the user and we will display this value afterwards:
# coding: utf-8
age = input ( "How old are you?:" )
print ( "You are% d years old" % age)
Note that if you are working with python 2.7, it is impossible to pass data other than numeric to the function input
, for python 2.7 we will prefer to use the function raw_input
which does much the same thing.
Comments in python
Whether you are alone developing your scripts or with others, it will always be essential to comment on your work. For example if you are creating a function that spans hundreds of lines of code, it will be more efficient to write a small description of your function above it rather than having to re-read all the code to understand this function. months later.
Comments in python begin with the sign #
Example:
# coding: utf-8
# This function asks a question to the user
# and this one must answer by a number obligatorily
age = input ( "How old are you?:" )
print ( "You are % d years old" % age )
Import features from other files
For the most ambitious projects, it will quickly be important to organize your work. The functions will multiply and it will be necessary to save them in separate files for more flexibility.
Let's create another file that we will name func.py
in the same folder as the file fiche.py
func.py
# coding: utf-8
def add_a ( v ):
return v + 1
fiche.py
# coding: utf-8
from func import *
age = input ( "How old are you?:" )
print ( "You are % d years old" % age )
age_plus_un = add_un ( age )
print ( "In a year you will be % d years old" % age_plus_un )
Instructions, functions, modules, packages
We have therefore seen that when we combine functions in a file, we create a set of functions which we call " module ".
When we seek to group modules together, we speak of a package .
Create a package
To create your own package, first create in the same folder as your program - a folder with the name of your package. In our example, we will name it " utils ".
In this folder, let's create the following file: __init__.py , this tells python that this is a package . This file can be empty, only its presence is important.
Then create a file still in this utils directory that we will name for example " operations.py
"
Content of your project file:
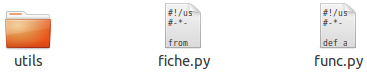
Contents of the dossier utils:
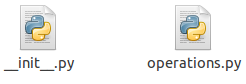
Now let's edit the file operations.py
and create a new function
# coding: utf-8
def add_two ( v ):
return v + 2
Then add a call to this function in the file fiche.py
# coding: utf-8
from func import *
from utils.operations import add_two
age = input ( "How old are you?:" )
print ( "You are % d years old" % age )
age_plus_un = add_un ( age )
print ( "In a year you will be % d years old" % age_plus_un )
age_plus_deux = add_two ( age )
print ( "In a year you will be % d years old" % age_plus_deux )
So what do we notice? First we import a package with the keywords from and import , then to call a specific function, we go through the following hierarchy:
from package.module import function
If you want to import all the functions of a module, you can indicate a star * which often means "ALL" in computer science.
Python modules
Here is a list of basic modules that you will have to use one day or another.
random: functions allowing to work with random values
math: all the functions useful for mathematical operations (cosine, sine, exp, etc.)
sys: system functions
os: functions allowing to interact with the operating system
time: functions for working over time
calendar: calendar functions
profile: functions used to analyze the execution of functions
urllib2: functions for retrieving information from the internet
re: functions allowing to work on regular expressions
Python file extensions
There are several file extensions that revolve around python:
.py -> editable script
.pyc -> compiled script
.pyw -> script executed without launching a terminal (under windows)